Missing topic notifications
The Diffusion server provides a feature to notify sessions about missing topic subscriptions. If a session registers itself as a handler for missing topics subscriptions, in a topic tree branch; then, the server will send notifications to such handlers, as soon as any other session subscribes using a topic selector that intersects the topic tree branch, but selects no existing topics. The handler can then do anything upon receiving such notification such as creating a topic at the notified path for which subscription occurred, or something else, as required.
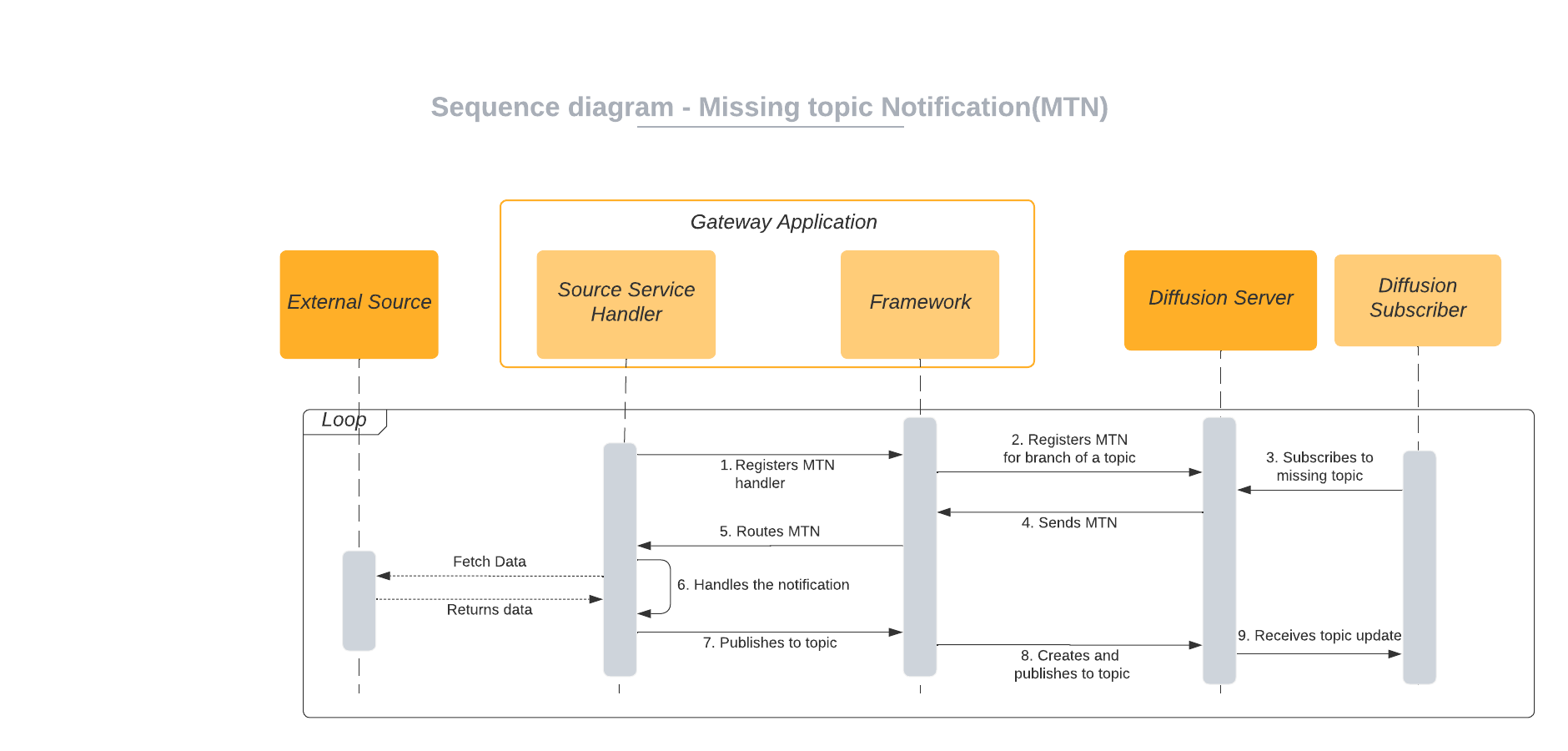
The Gateway Framework supports the use of this feature with source services. A source service handler can register a handler to be notified of missing topic notifications for a specified topic tree branch. The handler can handle the notification as required.
Handling missing topic notifications
To register for missing topic notifications, the Publisher.addMissingTopicHandler
method should be used.
This method requires:
-
a topicPath - which specifies a branch of a topic tree, for which the service should receive notifications, and
-
a
MissingTopicNotificationHandler
- which receives the notification, if any other session subscribes to a topic selector (matching the topic branch), which matches no existing topics in the server.
In the example of MissingTopicNotificationHandler implementation, a MissingTopicNotificationHandler is registered in the start method.
@Override
public CompletableFuture<?> start() {
return publisher
.addMissingTopicHandler(
missingTopicSelector,
missingTopicNotificationHandler);
}
Example: Registering a missing topic notification handler with the publisher
Below is the sample implementation of MissingTopicNotificationHandler
presented in the example. Here, on receiving the missing topic notification in onMissingTopic method, the publisher instance is used to publish to the missing topic.
private static final class MissingTopicNotificationHandlerImpl implements MissingTopicNotificationHandler {
private final Publisher publisher;
private MissingTopicNotificationHandlerImpl(Publisher publisher) {
this.publisher = publisher;
}
@Override
public CompletableFuture<?> onMissingTopic(
MissingTopicNotification missingTopicNotification) {
final String missingTopicPath =
missingTopicNotification.getTopicPath();
LOG.info("{} topic is missing. Publishing to this topic",
missingTopicPath);
try {
publisher.publish(
missingTopicPath,
"{\n" +
" \"dummy\": \"data\"\n" +
"}")
.whenComplete((result, ex) -> {
if (ex != null) {
LOG.error(
"Failed to publish to {} topic",
missingTopicPath);
}
});
}
catch (PayloadConversionException ex) {
throw new IllegalStateException(ex);
}
return CompletableFuture.completedFuture(null);
}
}
Multiple source services types can register multiple missing topic notification handlers for same topic path. In such cases, the Framework will route the notification to all registered handlers. It is up to the application to decide how to, or even whether, to handle the notification.
When a service is paused, the handler will not get any notifications, even if the application is still connected to the server, and the server sends notifications to the Framework.
The received notifications will be stored in an internal queue. If the service is resumed the list of notifications will be processed. This ensures that the notifications are not lost when a service is paused by a user, or due to application error.
On the other hand, it also means that the notifications could be stale, if a service is paused for a long time. This should be considered when handling the notifications.